视频链接:https://www.bilibili.com/video/av76382561/
项目地址:https://github.com/liangwj45/Unity-3D/tree/master/HW9/
游戏设计要求
游戏截图
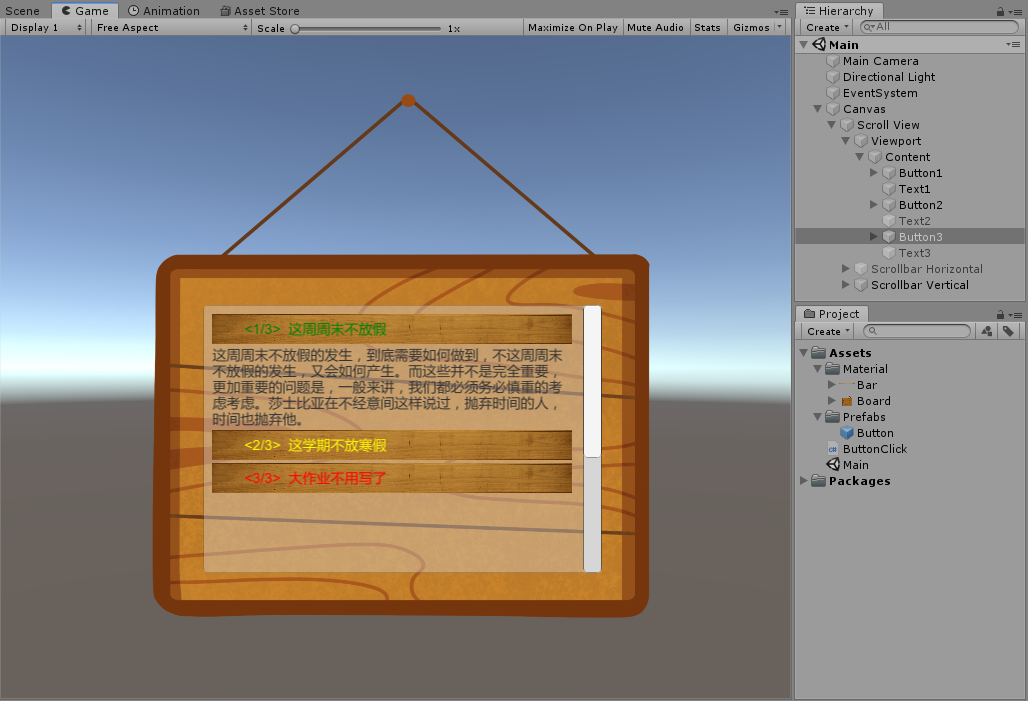
制作预制
制作一个 Button 的预制,主要是设置按钮的背景图片和其 Text 子对象的文字格式(左对齐),以及方便后面统一绑定代码定制相同的点击行为。
设计场景
先创建一个 Scroll View,然后在 Content 下创建 3 个 Button(使用预制的 Button)和 3 个 Text。(见上图)
给 Canvas 对象添加一个 Image 组件,并为其设置背景图片。

调整 Scroll View 对象和 Content 对象的宽度和高度。编写所有 Text 的内容,可以使用 html 的格式修改文字颜色。
编写代码
参考了师兄的代码后知道,原来可以使用协程来实现动画,那太棒了,所以自己也来尝试一下。
代码主要是控制文本框的行为,通过按钮的点击来控制文本框的展开和关闭。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76
| using System.Collections; using UnityEngine; using UnityEngine.UI;
public class ButtonClick : MonoBehaviour {
public Text text; private int frame = 20; private float text_width = 0;
void Start() { gameObject.GetComponent<Button>().onClick.AddListener(ActionOnClick); text_width = text.rectTransform.sizeDelta.x; }
void ActionOnClick() { if (text.gameObject.activeSelf) { StartCoroutine(CloseText()); } else { StartCoroutine(OpenText()); } }
private IEnumerator CloseText() { float rotation_x = 0; float rotate_speed = 90f / frame;
float text_height = 80f; float scale_speedh = text_height / frame;
for (int i = 0; i < frame; i++) { rotation_x -= rotate_speed; text_height -= scale_speedh; text.transform.rotation = Quaternion.Euler(rotation_x, 0, 0); text.rectTransform.sizeDelta = new Vector2(text_width, text_height); if (i == frame - 1) { text.gameObject.SetActive(false); } yield return null; } }
private IEnumerator OpenText() { float rotation_x = -90; float rotate_speed = 90f / frame;
float text_height = 0; float scale_speedh = 80f / frame;
for (int i = 0; i < frame; i++) { rotation_x += rotate_speed; text_height += scale_speedh; text.transform.rotation = Quaternion.Euler(rotation_x, 0, 0); text.rectTransform.sizeDelta = new Vector2(text_width, text_height); if (i == 0) { text.gameObject.SetActive(true); } yield return null; } } }
|